- 14 Tháng năm 2017
- 3,974
- 7,619
- 744
- 21
- Phú Yên
- Trường THPT Lương Văn Chánh


Ngồi cả buổi tối chỉ vì cái này. Code viết quá phức tạp luôn nhưng có chơi là được roài nha 
Dùng phím bấm để xoay nha.....
Bấm Enter để cho nó tự Random.
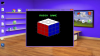
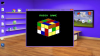
@ka1412 , @Nguyễn Tùng Ân , @son_gohan , @Tạ Đặng Vĩnh Phúc
Mã:
Program Rubik;
uses crt,graph;
const
Size = 3;
Color: array[1..6] of Word = (Red,Blue,Brown,Green,White,Yellow);
key: array[1..3,1..2*Size] of char =
(('q','w','e','r','t','y'),('a','s','d','f','g','h'),
('z','x','c','v','b','n'));
var gd,gm: integer;
Cube: array[1..6,1..Size,1..Size] of Word;
i,j,l: integer;
k: char;
Procedure CreateCube;
var i,x,y: integer;
Begin
for i := 1 to 6 do
for x := 1 to Size do
for y := 1 to Size do
cube[i,x,y] := Color[i];
End;
Procedure DrawKey;
var i: integer;
Begin
SetColor(9);
for i := 1 to Size do
begin
OutTextxy(125+i*50,300+i*17,key[1,i+Size]);
OutTextxy(280+i*50,80+i*17,key[1,i]);
OutTextxy(270+i*50,370-i*17,key[2,i+Size]);
OutTextxy(120+i*50,140-i*17,key[2,i]);
OutTextxy(130,125+i*50,key[3,i+Size]);
OutTextxy(465,125+i*50,key[3,i]);
end;
End;
Procedure DrawCube;
Begin
SetColor(DarkGray);
SetLineStyle(0,0,3);
{ UP }
Line(300,200,150,150);
Line(300,200,450,150);
Line(300,100,150,150);
Line(300,100,450,150);
Line(250,183,400,133);
Line(200,166,350,116);
Line(350,183,200,133);
Line(400,166,250,116);
{Left}
Line(300,200,300,350);
Line(150,150,150,300);
Line(150,300,300,350);
Line(250,183,250,333);
Line(200,166,200,316);
Line(300,250,150,200);
Line(300,300,150,250);
{Right}
Line(450,150,450,300);
Line(450,300,300,350);
Line(350,183,350,333);
Line(400,166,400,316);
Line(300,250,450,200);
Line(300,300,450,250);
End;
Procedure DrawColor;
var x,y: integer;
Begin
for x := 1 to Size do
for y := 1 to Size do
begin
SetFillStyle(1,Cube[1,x,y]);
FloodFill(150+(x-1)*50+25,150+(x-1)*17+(y-1)*50+25,DarkGray);
SetFillStyle(1,Cube[2,x,y]);
FloodFill(300+(x-1)*50+25,200-(x-1)*17+(y-1)*50+25,DarkGray);
SetFillStyle(1,Cube[5,x,y]);
FloodFill(150+(y-1)*50+(x-1)*50+25,150-(x-1)*17+(y-1)*17,DarkGray);
end;
End;
Procedure Rotate(k: char);
var x,y,i,j: integer;
tam: word;
Begin
case k of
'a','s','d':
begin
case k of
'a': j := 1;
's': j := 2;
'd': j := 3;
end;
for y := 1 to Size do
begin
tam := Cube[5,j,y];
Cube[5,j,y] := Cube[2,j,y];
Cube[2,j,y] := Cube[6,j,y];
Cube[6,j,y] := Cube[4,4-j,4-y];
Cube[4,4-j,4-y] := Tam;
end;
if (k = 'a') then
begin
j := 1;
tam := Cube[j,1,1];
Cube[j,1,1] := Cube[j,3,1];
Cube[j,3,1] := Cube[j,3,3];
Cube[j,3,3] := Cube[j,1,3];
Cube[j,1,3] := tam;
tam := Cube[j,2,1];
Cube[j,2,1] := Cube[j,3,2];
Cube[j,3,2] := Cube[j,2,3];
Cube[j,2,3] := Cube[j,1,2];
Cube[j,1,2] := tam;
end
else if k = 'd' then
begin
j := 3;
tam := Cube[j,1,1];
Cube[j,1,1] := Cube[j,1,3];
Cube[j,1,3] := Cube[j,3,3];
Cube[j,3,3] := Cube[j,3,1];
Cube[j,3,1] := tam;
tam := Cube[j,2,1];
Cube[j,2,1] := Cube[j,1,2];
Cube[j,1,2] := Cube[j,2,3];
Cube[j,2,3] := Cube[j,3,2];
Cube[j,3,2] := tam;
end;
end;
'z','x','c':
begin
case k of
'z': j := 1;
'x': j := 2;
'c': j := 3;
end;
for x := 1 to Size do
begin
tam := Cube[4,x,j];
Cube[4,x,j] := Cube[3,x,j];
Cube[3,x,j] := Cube[2,x,j];
Cube[2,x,j] := Cube[1,x,j];
Cube[1,x,j] := Tam;
end;
if (k = 'z') then
begin
j := 5;
tam := Cube[j,1,1];
Cube[j,1,1] := Cube[j,3,1];
Cube[j,3,1] := Cube[j,3,3];
Cube[j,3,3] := Cube[j,1,3];
Cube[j,1,3] := tam;
tam := Cube[j,2,1];
Cube[j,2,1] := Cube[j,3,2];
Cube[j,3,2] := Cube[j,2,3];
Cube[j,2,3] := Cube[j,1,2];
Cube[j,1,2] := tam;
end
else if k = 'c' then
begin
j := 6;
tam := Cube[j,1,1];
Cube[j,1,1] := Cube[j,1,3];
Cube[j,1,3] := Cube[j,3,3];
Cube[j,3,3] := Cube[j,3,1];
Cube[j,3,1] := tam;
tam := Cube[j,2,1];
Cube[j,2,1] := Cube[j,1,2];
Cube[j,1,2] := Cube[j,2,3];
Cube[j,2,3] := Cube[j,3,2];
Cube[j,3,2] := tam;
end;
end;
'q','w','e':
begin
case k of
'q': j := 1;
'w': j := 2;
'e': j := 3;
end;
for x := 1 to Size do
begin
tam := Cube[5,x,j];
Cube[5,x,j] := Cube[1,j,4-x];
Cube[1,j,4-x] := Cube[6,4-x,4-j];
Cube[6,4-x,4-j] := Cube[3,4-j,x];
Cube[3,4-j,x] := Tam;
end;
if (k = 'q') then
begin
j := 4;
tam := Cube[j,1,1];
Cube[j,1,1] := Cube[j,3,1];
Cube[j,3,1] := Cube[j,3,3];
Cube[j,3,3] := Cube[j,1,3];
Cube[j,1,3] := tam;
tam := Cube[j,2,1];
Cube[j,2,1] := Cube[j,3,2];
Cube[j,3,2] := Cube[j,2,3];
Cube[j,2,3] := Cube[j,1,2];
Cube[j,1,2] := tam;
end
else if k = 'e' then
begin
j := 2;
tam := Cube[j,1,1];
Cube[j,1,1] := Cube[j,1,3];
Cube[j,1,3] := Cube[j,3,3];
Cube[j,3,3] := Cube[j,3,1];
Cube[j,3,1] := tam;
tam := Cube[j,2,1];
Cube[j,2,1] := Cube[j,1,2];
Cube[j,1,2] := Cube[j,2,3];
Cube[j,2,3] := Cube[j,3,2];
Cube[j,3,2] := tam;
end;
end;
end;
End;
Procedure StartGame;
var i,j,k: integer;
Begin
for i := 1 to 20 do
begin
k := 1 + Random(Size);
j := 1 + Random(2*Size);
Rotate(key[k,j]);
DrawColor;
Delay(100);
end;
End;
BEGIN
InitGraph(gd,gm,'');
Randomize;
CreateCube;
DrawCube;
DrawKey;
DrawColor;
SetTextStyle(0,0,3);
SetColor(LightGreen);
OutTextXy(180,50,'RUBIK GAME');
repeat
k := #0;
if Keypressed then begin k := readkey;end;
if k = #13 then StartGame else
for i := 1 to Size do
for j := 1 to Size do
if k = key[i,j] then begin Rotate(k);DrawColor;end
else if k = key[i,j+Size] then
begin
for l := 1 to 3 do Rotate(key[i,j]);
DrawColor;
end;
until k =#27;
CloseGraph;
END.
Dùng phím bấm để xoay nha.....
Bấm Enter để cho nó tự Random.
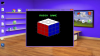
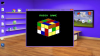
@ka1412 , @Nguyễn Tùng Ân , @son_gohan , @Tạ Đặng Vĩnh Phúc
Last edited: